Refs #5464 - FileFinder.findRuns() to throw on missing file.
Appropriate changes made to files that call the function, to ensure we still report or ignore missing files in the same manner as done previously.
- Loading branch information
- +9 −1 Code/Mantid/Framework/API/src/FileFinder.cpp
- +14 −0 Code/Mantid/Framework/API/test/FileFinderTest.h
- +5 −3 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/OSIRISDiffractionReduction.py
- +5 −6 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/RefEstimates.py
- +10 −13 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/RefLReduction.py
- +4 −5 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/RefLview.py
- +0 −6 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/RetrieveRunInfo.py
- +4 −4 Code/Mantid/Framework/PythonAPI/PythonAlgorithms/sfCalculator.py
- +6 −2 Code/Mantid/Framework/WorkflowAlgorithms/src/RefReduction.cpp
- +3 −4 Code/Mantid/scripts/Inelastic/CommonFunctions.py
- +24 −18 Code/Mantid/scripts/Interface/reduction_gui/widgets/reflectometer/base_ref_reduction.py
- +14 −10 Code/Mantid/scripts/Interface/reduction_gui/widgets/reflectometer/refl_data_simple.py
- +20 −14 Code/Mantid/scripts/Interface/reduction_gui/widgets/reflectometer/refl_sf_calculator.py
- +14 −10 Code/Mantid/scripts/Interface/reduction_gui/widgets/reflectometer/refm_reduction.py
- +4 −4 Code/Mantid/scripts/LargeScaleStructures/ScalingFactorCalculation/sfCalculator.py
- +6 −3 Code/Mantid/scripts/reduction/instruments/reflectometer/data_manipulation.py
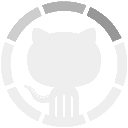